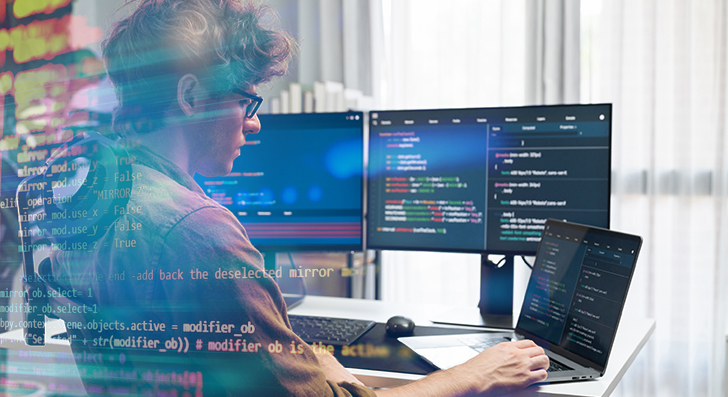
Scalability indicates your software can take care of development—much more buyers, additional info, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your prepare from the start. Quite a few programs are unsuccessful after they develop speedy due to the fact the original style and design can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your method will behave stressed.
Start by planning your architecture to be flexible. Prevent monolithic codebases exactly where almost everything is tightly related. As an alternative, use modular style and design or microservices. These patterns break your app into scaled-down, unbiased components. Just about every module or service can scale on its own without having influencing The complete method.
Also, take into consideration your databases from day 1. Will it need to have to take care of one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than current circumstances. Take into consideration what would come about If the consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like message queues or party-pushed devices. These enable your app handle more requests with out getting overloaded.
When you build with scalability in your mind, you are not just getting ready for success—you're reducing upcoming complications. A properly-planned method is easier to take care of, adapt, and increase. It’s far better to organize early than to rebuild later on.
Use the correct Database
Deciding on the appropriate database is a critical Section of creating scalable applications. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your app grows.
Start out by comprehension your information. Is it remarkably structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to manage much more website traffic and information.
If the info is a lot more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing large volumes of unstructured or semi-structured knowledge and can scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a large produce load? Look into databases that will cope with high compose throughput, as well as party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Consider forward. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you received’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And constantly keep an eye on databases effectiveness when you improve.
To put it briefly, the ideal databases relies on your application’s framework, pace wants, And the way you count on it to expand. Choose time to select sensibly—it’ll help save many difficulties later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by creating clean, very simple code. Keep away from repeating logic and remove anything unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular functions. Keep the features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of a lot of memory.
Up coming, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every question only asks for the data you really have to have. Keep away from SELECT *, which fetches almost everything, and instead decide on specific fields. Use indexes to speed up lookups. And stay clear of performing a lot of joins, especially across substantial tables.
In the event you detect the same knowledge remaining requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t really have to repeat expensive operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to take a look at with substantial datasets. Code and queries that work good with one hundred data could possibly crash when they have to handle 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more site visitors. If every little thing goes by means of one particular server, it is going to speedily turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused promptly. When end users request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database website anytime. You'll be able to provide it through the cache.
There are two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers knowledge in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers databases load, enhances velocity, and helps make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you would like each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess potential capability. When targeted traffic boosts, you may increase more resources with just a few clicks or immediately making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply companies like managed databases, storage, load balancing, and safety equipment. You'll be able to center on making your application as an alternative to controlling infrastructure.
Containers are Yet another crucial Instrument. A container packages your application and all the things it ought to operate—code, libraries, options—into a single unit. This can make it quick to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses multiple containers, instruments like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
To put it briefly, employing cloud and container tools suggests you are able to scale speedy, deploy very easily, and Get better swiftly when complications come about. If you want your app to mature without having restrictions, commence working with these resources early. They help save time, reduce threat, and assist you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a essential Component of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how long it takes for customers to load webpages, how often problems take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This helps you fix issues speedy, normally in advance of people even observe.
Monitoring is also practical after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, it is possible to roll it back again prior to it results in authentic hurt.
As your app grows, targeted visitors and facts boost. With out checking, you’ll overlook signs of hassle right up until it’s as well late. But with the ideal instruments in place, you continue to be in control.
To put it briefly, checking helps you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works properly, even stressed.
Ultimate Thoughts
Scalability isn’t only for big firms. Even little applications require a solid foundation. By planning cautiously, optimizing correctly, and using the appropriate tools, it is possible to build apps that mature smoothly devoid of breaking stressed. Commence smaller, Believe massive, and build wise.